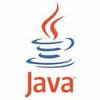
1. What is java
Java is:
• A new Object Oriented Programming Language developed at Sun Microsystems.
• Easier to learn than most other Object Oriented programming languages, since it has collected the best parts of the existing ones.
• A language that is standardized enough so that executable applications can run on any (in principle) computer that contains a Virtual Machine (run-time environment).
• Virtual machines can be embedded in web browsers (such as Netscape Navigator, Microsoft Internet Explorer, and IBM WebExplorer) and operating systems.
• A standardized set of Class Libraries (packages), that support creating graphical user interfaces, controlling multimedia data and communicating over networks.
• A programming language that supports the most recent features in computer science at the programming language level.
Java is not:
• Slower than any other Object Oriented language. Java applications can always be compiled to native code and run as fast as any other program.
• 100% compatible yet, since the same application does not always look the same on all platforms due to virtual machine implementation bugs and differences.
1.1 Different kinds of Java applications
Java applications can be of several kinds. The three main ones treated here are:
• Programs with a textual interface.
• Applets used in Internet browsers.
• Windowing applications that use a graphical user interface.
These three kinds of application may all easily be included in the same Java program as can be shown by the following examples.
Introduction to Java programming K.Främling Page 6
Example 1. Simple text interface application (compiled and run)
publicclassHelloWorld
{
publicstaticvoidmain(Stringargv[])
{
System.out.println("Hello,world!");
}
}
The first line “publicclassHelloWorld” defines a “class” that is called HelloWorld. Java is a pure object oriented language, so everything has to be contained in classes (no global functions like in “C” or C++).
”publicstaticvoidmain(Stringargv[])” is the standard declaration for a program entry point in Java. Any class may contain a ”main” function, so the same program may have several program entry points. This is useful for test and debugging purposes.
”System.out.println("Hello,world!");” just writes out the text that we want onto the standard output. ”System” is a pre-defined class that contains the member ”out”, which is the object that corresponds to the standard output. ”println” is a method of this object that takes the text to write out as a parameter. The resulting output is the given text followed by a newline character. These concepts will be explained in detail further on.
To compile this program, we first save it as a file named ”HelloWorld.java”. In fact, the file name always has to be the same as the class name for a public class and it has to have the extension ”.java”.
Then, if we are in the same directory as the saved file, we can compile it with the DOS command ”javacHelloWorld.java” (if the Java development environment is set up correctly).
We run it with the command line ”javaHelloWorld” (if we are still in the same directory and the Java environment is set up correctly).
Introduction to Java programming K.Främling Page 7
Example 2. Simple applet (compiled and run)
importjava.awt.*;
importjava.applet.Applet;
publicclassHelloWorldAppletextendsApplet
{
publicvoidpaint(Graphicsg)
{
g.drawString("Hello,world!",0,25);
}
}
The corresponding HTML page looks like this:
<html>
<head>
<title>HelloWorldExamplefortheJavaBasicsCourse</title>
</head>
<body>
<h1>HelloWorldExamplefortheJavaBasicsCourse</h1>
<hr>
<appletcode=HelloWorldApplet.classwidth=100height=50>
alt="Yourbrowserunderstandsthe<APPLET>tagbutisn'trunningtheapplet,forsomereason."
Yourbrowseriscompletelyignoringthe<APPLET>tag!
</applet>
<hr>
<ahref="HelloWorldApplet.java">Thesource</a>.
</body>
</html>
Example 2 shows a very simple applet, that just writes out “Hello,world!” in the HTML page shown. The resulting page is shown in Figure 1.
The first two lines, “importjava.awt.*;” and “importjava.applet.Applet;” are for including external libraries, just like “.h”-files in “C” and C++.
Then we define the class “HelloWorldApplet” that is a derived class from the Applet class, which is indicated by the words “extendsApplet” at the end of the line. A derived class is a class that inherits from the behaviour of its’ base class. The Applet class already contains most of the program code for executing the applet, so we only need to redefine the behaviour that we need. In this case, we only override the method “publicvoidpaint(Graphicsg)” with our own behaviour.
“g.drawString("Hello,world!",0,25);” draws the given string at the given position of the applet display area.
Introduction to Java programming K.Främling Page 8
Figure 1. Simple applet screen.
The applet is included in an HTML page using the ”applet” tag. It takes a number of arguments, which are:
• code: name of the “.class” file that contains the compiled applet.
• width, height: width and height of the display area reserved for the applet.
• alt: a text to show if the browser is not capable of executing the applet.
Applet display is only possible within the display area that has been reserved for it. An applet may, however, tell the browser to go to a new page, for instance.
Introduction to Java programming K.Främling Page 9
Example 3. Simple “app-applet” (compiled and run)
importjava.awt.*;
importjava.applet.Applet;
publicclassHelloWorldFrameextendsApplet
{
publicstaticvoidmain(Stringargv[])
{
Framef;
f=newFrame();
f.setLayout(newGridLayout());
f.add(newHelloWorldFrame());
f.setSize(100,100);
f.show();
}
publicvoidpaint(Graphicsg)
{
g.drawString("Hello,world!",0,25);
}
}
Example 3 shows how to make the applet executable as an independent application (i.e. without any need for an Internet browser). The trick is to create a normal program entry point, which creates a window (a “Frame” object) that the applet can be displayed in.
“Framef;” declares a variable f that is a reference to a Frame object. “f=newFrame();” creates a new “Frame” object and makes “f” reference it. The following lines set up some characteristics of the frame, adds the “HelloWorldFrame” applet to it and then displays the frame.
This kind of an application is often called an “app-applet” since it may be included as an applet in a HTML page or it may be run as an independent application. If no applet behaviour is needed, then the “HelloWorldFrame” could be derived from the “Panel” base class, for instance.
1.2 Platform independence
Platform independence means that the same (compiled) program can be executed on any computer.
It is obvious that this is necessary in Internet applications, since any kind of machine may be used to see WWW pages. This is why most recent Internet browsers include a Java Virtual Machine (JVM) that executes instructions generated by a Java compiler.
Introduction to Java programming K.Främling Page 10
This Java virtual machine can also be incorporated or embedded into the kernel of the operating system. Java virtual machines nowadays exist for most operating systems.
We call the JVM a virtual machine because the Java compiler produces compiled code whose target machine is this virtual machine instead of being a physical processor or computer. A Java program is said to be compiled to byte-codes, whose target architecture is the Java Virtual Machine, as illustrated by Figure 2.
Figure 2. Compilation of a Java program to byte-codes and executing it with the Java VM.
Figure 2 also shows the development process a typical Java programmer uses to produce byte codes and execute them. The first box indicates that the Java source code is located in a “.java” file, which is processed with a Java compiler called “javac”. The Java compiler produces a file called a “.class” file, which contains the byte code. The “.class” file is then loaded across the network or loaded locally on your machine into the execution environment on that platform. Inside that environment is the Java virtual machine, which interprets and executes the byte code.
1.3 History of Java
1990 – 1993: James Gosling and others at Sun were working on a project that needed a new programming language. Their project was called *7 (Star Seven) and its’ goal was developing a universal system for small, personal digital assistance in set top boxes.
”Oak” was the original name of the language developed, named after the tree outside James Gosling's office. But Oak turned out to be an existing programming language, so Sun named it Java, after many visits to a local coffee shop! Java is not an acronym. It is a trademark of Sun Microsystems.
1993 – 1995: World Wide Web (WWW) emerged and made Sun develop a web browser that was originally named WebRunner and later HotJava. HotJava was presented to various companies, including Netscape Communications, who incorporated it in their product.
1995 – 1996: Companies begin to license the Java technology and to incorporate it into their products.
Introduction to Java programming K.Främling Page 11
1997 - : Java becomes increasingly popular and most operating systems provide a Java Virtual Machine. Java begins to be used for application develoment, not only for applets. Microsoft understands the threat that Java represents and tries to ”pollute” the language in order to take it over.
Java was already from the start meant to be multi-platform. It was also intended to be very light-weight, especially concerning the size of the compiled programs and the size of the virtual machine. These are the main reasons for its’ suitability for WWW applications.
Java shares many characteristics of the two most important existing object oriented programming languages, which are Smalltalk and C++. Characteristics common with Smalltalk are:
• Similar object model (single-rooted inheritance) hierarchy, access to objects via reference only, ...).
• Compiled to byte-code (initially interpreted).
• Dynamic memory layout plus garbage collection.
• Simple inheritance.
Common characteristics with C++ are:
• Same syntax for expressions, statements, and control flow.
• Similar OO structural syntax (classes, access protection, constructors, method declaration, ...).
What makes Java more interesting than both these languages is that it has borrowed all the best ideas in Smalltalk and avoided the bad ideas in C++, which make C++ a very difficult language to learn.
Java is still a very young language. This is the reason why there exists several specification levels of the language:
• 1.0 - 1.0.2: Appeared in 1995. Contained the complete specification of the language and a class library for the most essential things.
• 1.1 – 1.1.7A: Appeared in 1997. Included improvements in the graphical interface and added most of the functionalities that are usually included in proprietary class libraries (MFC, OWL, …). Networking was also improved, especially for distributed computing.
Introduction to Java programming K.Främling Page 12
Java takes a direction where it provides all the functionalities that are normally provided by the operating system. The Java virtual machines become very rapid by version 1.1.7A. Java Beans are specified and supported.
• Java 2: Appeared in December 1998. New interface libraries and several improvements, especially in handling multimedia and distributed computing. Unfortunately, this version seems to be very slow (at least the beta versions tested).
Most of the contents of this course stay within the limits of the 1.0.2 specification, since most Internet browsers have updated to 1.1 specifications only recently.
FOR MORE INFORMATION VISIT THE FOLLOWING LINKS
http://gsb.haifa.ac.il/~sheizaf/ecommerce/java.lecture.html
http://java.sun.com/developer/technicalArticles/ALT/Reflection/
http://www.roseindia.net/java/use-java/uses-of-java.shtml
http://www.google.com/url?sa=t&source=web&cd=2&ved=0CCEQFjAB&url=http%3A%2F%2Fciteseerx.ist.psu.edu%2Fviewdoc%2Fdownload%3Fdoi%3D10.1.1.167.9827%26rep%3Drep1%26type%3Dpdf&rct=j&q=java%20inntroduction%20pdf&ei=bpAyTsv9PIPOrQf2sJDMCw&usg=AFQjCNFPuOYGkWrzVUvVEtPQnUbBY5rPfQ&sig2=vjVutd6inLWkeN-w-UKzzQ&cad=rja
http://math.hws.edu/javanotes/
http://appletree.or.kr/quick_reference_cards/Java-
JSP/DZone%20-%20Core%20Java.pdf
http://nisearch.com/search/pdf/advanced+java+complete+reference+pdf+free+download
http://www.blazonry.com/java/free-java-books-advanced.php
http://core-java-pdf.fyxm.net/
http://appletree.or.kr/quick_reference_cards/Java-
Features of .NET
Now that we know some basics of .NET, let us see what makes .NET a wonderful
platform for developing modern applications.
• Rich Functionality out of the box
.NET framework provides a rich set of functionality out of the box. It contains
hundreds of classes that provide variety of functionality ready to use in your
applications. This means that as a developer you need not go into low level details
of many operations such as file IO, network communication and so on.
• Easy development of web applications
ASP.NET is a technology available on .NET platform for developing dynamic
and data driven web applications. ASP.NET provides an event driven
programming model (similar to Visual Basic 6 that simplify development of web
pages (now called as web forms) with complex user interface. ASP.NET server
controls provide advanced user interface elements (like calendar and grids) that
save lot of coding from programmer’s side.
• OOPs Support
The advantages of Object Oriented programming are well known. .NET provides
a fully object oriented environment. The philosophy of .NET is – “Object is
mother of all.” Languages like Visual Basic.NET now support many of the OO
features that were lacking traditionally. Even primitive types like integer and
characters can be treated as objects – something not available even in OO
languages like C++.
• Multi-Language Support
Generally enterprises have varying skill sets. For example, a company might have
people with skills in Visual Basic, C++, and Java etc. It is an experience that
whenever a new language or environment is invented existing skills are outdated.
This naturally increases cost of training and learning curve. .NET provides
something attractive in this area. It supports multiple languages. This means that
if you have skills in C++, you need not throw them but just mould them to suit
.NET environment. Currently four languages are available right out of the box
namely – Visual Basic.NET, C# (pronounced as C-sharp), Jscript.NET and
Managed C++ (a dialect of Visual C++). There are many vendors that are
working on developing language compilers for other languages (20+ language
compilers are already available). The beauty of multi language support lies in the
fact that even though the syntax of each language is different, the basic
capabilities of each language remain at par with one another.
• Multi-Device Support
Modern lift style is increasingly embracing mobile and wireless devices such as
PDAs, mobiles and handheld PCs. . . .NET provides promising platform for
programming such devices. .NET Compact Framework and Mobile Internet
Toolkit are step ahead in this direction.
• Automatic memory management
While developing applications developers had to develop an eye on system
resources like memory. Memory leaks were major reason in failure of
applications. .NET takes this worry away from developer by handling memory on
its own. The garbage collector takes care of freeing unused objects at appropriate
intervals.
• Compatibility with COM and COM+
Before the introduction of .NET, COM was the de-facto standard for
componentized software development. Companies have invested lot of money and
efforts in developing COM components and controls. The good news is – you can
still use COM components and ActiveX controls under .NET. This allows you to
use your existing investment in .NET applications. .NET still relies on COM+ for
features like transaction management and object pooling. In fact it provides
enhanced declarative support for configuring COM+ application right from your
source code. Your COM+ knowledge still remains as a valuable asset.
• No more DLL Hell
If you have worked with COM components, you probably are aware of “DLL
hell”. DLL conflicts are a common fact in COM world. The main reason behind
this was the philosophy of COM – “one version of component across machine”.
Also, COM components require registration in the system registry. .NET ends this
DLL hell by allowing applications to use their own copy of dependent DLLs.
Also, .NET components do not require any kind of registration in system registry.
• Strong XML support
Now days it is hard to find a programmer who is unaware of XML. XML has
gained such a strong industry support that almost all the vendors have released
some kind of upgrades or patches to their existing software to make it “XML
compatible”. Currently, .NET is the only platform that has built with XML right
into the core framework. .NET tries to harness power of XML in every possible
way. In addition to providing support for manipulating and transforming XML
documents, .NET provides XML web services that are based on standards like
HTTP, XML and SOAP.
• Ease of deployment and configuration
Deploying windows applications especially that used COM components were
always been a tedious task. Since .NET does not require any registration as such,
much of the deployment is simplified. This makes XCOPY deployment viable.
Configuration is another area where .NET – especially ASP.NET – shines over
traditional languages. The configuration is done via special files having special
XML vocabulary. Since, most of the configuration is done via configuration files,
there is no need to sit in front of actual machine and configure the application
manually. This is more important for web applications; simply FTPing new
configuration file makes necessary changes.
• Security
Windows platform was always criticized for poor security mechanisms. Microsoft
has taken great efforts to make .NET platform safe and secure for enterprise
applications. Features such as type safety, code access security and role based
authentication make overall application more robust and secure.
1.4 Installing the .NET Framework SDK
Now that you have fare idea of what .NET I and what it can do for you, it is time to
install .NET framework SDK on your machine. Following sections will tell you
everything you need to know for installing .NET framework.
• Hardware Requirements
In order to install .NET framework SDK following hardware is required:
- Computer/Processor : Intel Pentium class, 133 megahertz (MHz) or higher
- Minimum RAM Requirements : 128 megabytes (MB) (256 MB or higher
recommended)
- Hard Disk :
o Hard disk space required to install: 600 MB
o Hard disk space required: 370 MB
- Display : Video: 800x600, 256 colors
- Input Device : Microsoft mouse or compatible pointing device
• Software Requirements
- Microsoft Internet Explorer 5.01 or later is required
- Microsoft Data Access Components 2.6 is also required (Microsoft Data
Access Components 2.7 is recommended)
- Operating System :
o Microsoft Windows® 2000, with the latest Windows service pack and
critical updates available from the Microsoft Security Web page
o Microsoft Windows XP – (Microsoft Windows XP Professional if you
want to run ASP.NET)
o Microsoft Windows NT® 4.0
Note: If you want to simply run .NET applications then you can also run them on
Microsoft Windows XP Home edition, Windows Millennium Edition (Windows
ME) and Windows 98.
Here are some URLs that you will find handy in making your system up-to-date
for above software requirements.
Internet Explorer 6 can be downloaded from
http://www.microsoft.com/windows/ie/downloads/ie6/default.asp
Microsoft Data Access Components 2.7 can be downloaded from
http://www.microsoft.com/data/download_270RTM.htm
Various Windows service packs and patches can be obtained from
http://www.microsoft.com/downloads/search.asp
• Where to get .NET Framework SDK
As mentioned earlier .NET framework SDK is freely downloadable from MSDN
site. Visit
http://msdn.microsoft.com/downloads/default.asp?url=/downloads/sample.asp?url
=/msdn-files/027/000/976/msdncompositedoc.xml and download it now.
The total download size is 137,363,456 bytes (approximately 131 Mb). For your
convenience Microsoft has provided multi-part version of the entire download. If
you are unable to download the SDK from MSDN web site, check out popular PC
magazines around. Many of them contain .NET Framework SDK on their
companion CD.
• Starting the installation
Note: If you already have a previous version of .NET installed on the machine
then it must first be uninstalled. Refer ReadMe files that ship with .NET
framework SDK. These files contain valuable information related to installation,
system requirements and trouble shooting.
In order to start the installation, you need to run the setup program that is
available with the download mentioned above. A wizard will guide you with
necessary process. It will also allow you to select various components of the
framework.
FOR MORE INFORMATION VISIT THE FOLLOWING LINKS
http://www.uop.edu.jo/download/PdfCourses/vb.net/dotNET%20Tutorial%20for%20Beginners%20(1).pdf
http://wpr.gruner.org/download/wpr13-DOT.NET.pdf
http://www.pisa.org.hk/event/dotnet_overview.pdf
http://www.cse.iitk.ac.in/users/aca/dotnet/lecture_slides/dotnet_lec1.pdfhttp://www.ecma-international.org/activities/Languages/Introduction%20to%20Csharp.pdf
http://media.wiley.com/product_data/excerpt/98/07645482/0764548298.pdf

Introduction
The PDF functions in PHP can create PDF files using the PDFlib library which was initially created by Thomas Merz and is now maintained by » PDFlib GmbH.
The documentation in this section is only meant to be an overview of the available functions in the PDFlib library and should not be considered an exhaustive reference. For the full and detailed explanation of each function, consult the PDFlib Reference Manual which is included in all PDFlib packages distributed by PDFlib GmbH. It provides a very good overview of what PDFlib is capable of doing and contains the most up-to-date documentation of all functions.
For a jump start we urge you to take a look at the programming samples which are contained in all PDFlib distribution packages. These samples demonstrate basic text, vector, and graphics output as well as higher-level functions, such as the PDF import facility (PDI).
All of the functions in PDFlib and the PHP module have identical function names and parameters. Unless configured otherwise, all lengths and coordinates are measured in PostScript points. There are generally 72 PostScript points to an inch, but this depends on the output resolution. Please see the PDFlib Reference Manual included in the PDFlib distribution for a more thorough explanation of the coordinate system used.
With version 6, PDFlib offers an object-oriented API for PHP 5 in addition to the function-oriented API for PHP 4. The main difference is the following:
In PHP 4, first a PDF resource has to be retrieved with a function call like
$p = PDF_new().
This PDF resource is used as the first parameter in all further function calls, such as in
PDF_begin_document($p, "", "").
In PHP 5 however, a PDFlib object is created with
$p = new PDFlib().
This object offers all PDFlib API functions as methods, e.g. as with
$p->begin_document("", "").
In addition, exceptions have been introduced in PHP 5 which are supported by PDFlib 6 and later as well.
http://www.hoboes.com/NetLife/PHP/
http://ezinearticles.com/?Top-Ten-PHP-Uses&id=2918388
http://php.net/manual/en/ref.pdf.php
http://www.tuttoaster.com/getting-started-with-pdf-generation-in-php/

http://download.cnet.com/Free-HTML-to-PDF-Converter/3000-18497_4-10691753.html
http://www.pdfonfly.com/

http://www.coolinterview.com/type.asp?iType=167
http://www.a2zinterviewquestions.com/
http://www.techfaq360.com/JDBC_interview_questions.jsp
http://humanresources.about.com/od/interviewing/tp/interview_questions.htm
http://www.pdftutorials.com/tutorials/oracle-interview-questions-and-answers+871.html

Chapter 1 INTRODUCTION.
The Structured Query Language, SQL is a query language which is used with relational databases. This chapter starts by describing some of the terms used in data processing and how they relate to SQL. The later part of this chapter describes relational databases and how SQL is used to query them.
1.1 "A Collection of Related Data": Databases and Database Management Systems.
Let's start from basics. What is a database? In very general terms, a database is a collection of related data. Notice the word related, this implies that the collection of letters on this page do not by themselves constitute a database. But if we think of them as a collection of letters arranged to form words, then they can be conceptualised as data in a database. Using similar reasoning, we can also say that a tome such as a telephone directory is also a database. It is a database first, because it is a collection of letters that form words and second, because it is an alphabetical listing of people's names, their addresses and their telephone numbers. How we think of a database depends on what use we want to make of the information that it contains.
So far, we have talked about a database in it's broadest sense. This very general definition is not what most people mean when they talk about a database. In this electronic age, the word database has become synonymous with the term "computerised database". Collins English Dictionary describes a database as "A store of a large amount of information, esp. in a form that can be handled by a computer." In this book, we will be dealing only with computerised databases. In keeping with popular trend though, we will be using the word database to refer to a computerised database.
A database (computerised database remember) by itself, is not much use. The data is stored electronically on the computer's disk in a format which we humans cannot read or understand directly. What we need is some way of accessing this data and converting it into a form which we do understand. This is the job of the database management system or DBMS for short. A DBMS is essentially a suite of programs that act as the interface between the human operator and the data held in the database. Using the DBMS, it is possible to retrieve useful information, update or delete obsolete information and add new information to the database. As well as data entry and retrieval, the DBMS plays an important role in maintaining the overall integrity of the data in the database. The simplest example of is ensuring that the values entered into the database conform to the data types that are specified. For example, in the telephone book database, the DBMS might have to ensure that each phone number entered conforms to a set format of XXX-XXXXXXX where X represents an integer.
1.2 "The Database as a Collection of Tables": Relational databases and SQL.
In the early days of computerised databases, all large database systems conformed to either the network data model or the hierarchical data model. We will not be discussing the technical details of these models except to say that they are quite complex and not very flexible. One of the main drawbacks of these databases was that in order to retrieve information, the user had to have an idea of where in the database the data was stored. This meant that data processing and information retrieval was a technical job which was beyond the ability of the average office manager. In those days life was simple. data processing staff were expected to prepared the annual or monthly or weekly reports and managers were expected to formulate and implement day to day business strategy according to the information contained in the reports. Computer literate executives were rare and DP staff with business sense were even more rare. This was the state of affairs before the advent of relational databases.
The relational data model was introduced in 1970, E. F. Codd, a research fellow working for IBM, in his article `A Relational Model of Data for Large Shared Databanks'. The relational database model represented the database as a collection of tables which related to one another.
Unlike network and hierarchical databases, the relational database is quite intuitive to use, with data organised into tables, columns and rows. An example of a relational database table is shown in Figure 1.1. We can see just by looking at Figure 1.1 what the table is. The table is a list of people's names and telephone numbers. It is similar to how we might go about the task of jotting down the phone numbers of some of our friends, in the back of our diary for example.
The relational data model consists of a number of intuitive concepts for storing any type of data in a database, along with a number of functions to manipulate the information.
NUM | SURNAME | FIRSTNAME | PHONE_NUMBER |
1 | Jones | Frank | 9635 |
2 | Bates | Norman | 8313 |
3 | Clark | Brian | 2917 |
4 | Stonehouse | Mark | 3692 |
5 | Warwick | Rita | 3487 |
Figure 1.1
The relational data model as proposed by Codd provided the basic concepts for a new database management system, the relational database management system (RDBMS). Soon after the relational model was defined, a number of relational database languages were developed and used for instructing the RDBMS. Structured Query Language being one of them.
The SQL language is so inextricably tied to relational database theory that it is impossible to discuss it without also discussing the relational data model. The next two sections briefly describe some of the concepts of this model.
We have already seen that a relational database stores data in tables. Each column of the table represent an attribute, SURNAME, FIRSTNAME, PHONE_NUMBER for example. Each row in the table is a record. In the table in Figure 1.1, each row is a record of one person. A single table with a column and row structure, does not represent a relational database. Technically, this is known as a flat file or card index type database. Relational databases have several tables with interrelating data. Suppose that the information in the table of Figure 1.1 is actually the list of people working in the company with their telephone extensions. Now we get an idea that this simple table is actually a small part of the overall database, the personnel database. Another table, such as the one in Figure 1.2. could contain additional details on the persons listed in the first table.
NUM | D_O_B | DEPT | GRADE |
2 | 12/10/63 | ENG | 4 |
5 | 07/05/50 | DESIGN | 7 |
3 | 03/11/45 | SALES | 9 |
1 | 09/03/73 | ENG | 2 |
1.2.2 The Primary key and the foreign Key.
Figure 1.2
The two tables described in the previous section and shown in Figures 1.1 and 1.2, now constitute a relational database. Of course, in a real personnel database, you would need to store a great deal more information and would thus need a lot more related tables.
Notice that the first column in each table is the NUM column. The information stored in NUM does not really have anything to do with the person's record. Why is it there? The reason is that NUM is used to uniquely identify each person's record. We could have used the person's name, but chances are that in a large company, there would be more than one person with the same name. NUM is known as the primary key for the table of Figure 1.1. For the table of Figure 1.2, where a primary key of another table is used to relate data, NUM is a called a foreign key.
The primary keys and foreign keys are a very important part of relational databases. They are the fields that relate tables to each other. In the table of Figure 1.2 for example, we know that the first record is for Norman Bates because the value for NUM is 2 and we can see from the table of Figure 1.1 that this is Norman Bates' record.
1.3 "Communicating to the DBMS what you want it to do": Introduction to the SQL language.
The Structured Query Language is a relational database language. By itself, SQL does not make a DBMS. It is just a medium which is used to as a means of communicating to the DBMS what you want it to do. SQL commands consist of english like statements which are used to query, insert, update and delete data. What we mean by `english like', is that SQL commands resemble english language sentences in their construction and use. This does not mean that you can type in something like "Pull up the figures for last quarter's sales" and expect SQL to understand your request. What it does mean is that SQL is a lot easier to learn and understand than most of the other computer languages.
SQL is sometimes referred to as a non-procedural database language. What this means is that when you issue an SQL command to retrieve data from a database, you do not have to explicitly tell SQL where to look for the data. It is enough just to tell SQL what data you want to be retrieved. The DBMS will take care of locating the information in the database. This is very useful because it means that users do not need to have any knowledge of where the data is and how to get at it. Procedural languages such as COBOL or Pascal and even older databases based on the network and hierarchical data models require that users specify what data to retrieve and also how to get at it. Most large corporate databases are held on several different computers in different parts of the building or even at different geographic locations. In such situations, the non-procedural nature of SQL makes flexible, ad hoc querying and data retrieval possible. Users can construct and execute an SQL query, look at the data retrieved, and change the query if needed all in a spontaneous manner. To perform similar queries using a procedural language such as COBOL would mean that you would have to create, compile and run one computer programs for each query.
Commercial database management systems allow SQL to be used in two distinct ways. First, SQL commands can be typed at the command line directly. The DBMS interprets and processes the SQL commands immediately, and any result rows that are retrieved are displayed. This method of SQL processing is called interactive SQL. The second method is called programmatic SQL. Here, SQL statements are embedded in a host language such as COBOL or C. SQL needs a host language because SQL is not really a complete computer programming language as such. It has no statements or constructs that allow a program to branch or loop. The host language provides the necessary looping and branching structures and the interface with the user, while SQL provides the statements to communicate with the DBMS.
1.4 "A Research Project Conducted by IBM": The history of SQL.
The origins of the SQL language date back to a research project conducted by IBM at their research laboratories in San Jose,
California in the early 1970s. The aim of the project was to develop an experimental RDBMS which would eventually lead to a marketable product. At that time, there was a lot of interest in the relational model for databases at the academic level, in conferences and seminars. IBM, which already had a large share of the commercial database market with hierarchical and network model DBMSs, realised quite quickly that the relational model would figure prominently in future database products.
The project at IBM's San Jose labs was started in 1974 and was named System R. A language called Sequel (for Structured English QUEry Language) was chosen as the relational database language for System R. In the project, Sequel was abbreviated to SQL. This is the reason why SQL is still generally pronounced as see-quel.
In the first phase of the System R project, researchers concentrated on developing a basic version of the RDBMS. The main aim at this stage was to verify that the theories of the relational model could be translated into a working, commercially viable product. This first phase was successfully completed by the end of 1975, and resulted in a rudimentary, single-user DBMS based on the relational model.
The subsequent phases of System R concentrated on further developing the DBMS from the first phase. Additional features were added, multi-user capability was implemented, and by 1978, a completed RDBMS was ready for user evaluation. The System R project was finally completed in 1979. During this time, the SQL language was modified and added to as the needs of the System R DBMS dictated.
The theoretical work of the System R project resulted in the development and release in 1981 of IBM's first commercial relational database management system. The product was called SQL/DS and ran under the DOS/VSE operating system environment. Two years later, IBM announced a version of SQL/DS for the VM/CMS operating system. In 1983, IBM released a second SQL based RDBMS called DB2, which ran under the MVS operating system. DB2 quickly gained widespread popularity and even today, versions of DB2 form the basis of many database systems found in large corporate data-centres.
During the development of System R and SQL/DS, other companies were also at work creating their own relational database management systems. Some of them, Oracle being a prime example, even implemented SQL as the relational database language for
their DBMSs concurrently with IBM.
Today, the SQL language has gained ANSI (American National Standards Institute) and ISO (International Standards Organization) certification. A version of SQL is available for almost any hardware platform from CRAY supercomputers to IBM PC microcomputers. In recent years, there has been a marked trend for software manufacturers to move away from proprietary database languages and settle on the SQL standard. The microcomputer platform especially has seen a proliferation of previously proprietary packages that have implemented SQL functionality. Even spreadsheet and word processing packages have added options which allow data to be sent to and retrieved from SQL based databases via a Local Area or a Wide Area network connection.
1.5 "SQL Commands Build Upon Themselves": Organization of this book.
After this introduction, this book first presents the SQL language in a nutshell. Subsequent chapters then focus on explaining each of the SQL command groups (the SELECT, the UPDATE, the CREATE etc) more fully. The reason for this method of presentation is that a lot of the SQL commands build upon themselves. For example, you cannot discuss the INSERT INTO with SELECT command without having knowledge of and understanding the SELECT statement itself. So where do you put the chapter on INSERT INTO with SELECT? You can't put it before the chapter on SELECT because as we've said, it requires the reader to have knowledge of the SELECT statement. You can't put it after the chapter on SELECT because the SELECT statement requires data to be input into the tables by using the INSERT statement. We have gone for the second option because it is a lot easier to take a leap of faith and believe that somehow the tables are already populated with data and use SELECT to query them rather than trying to understand the INSERT INTO with SELECT without any knowledge of how SELECT works.
To save having to put phrases such as "see the later chapter on SELECT" or "see the earlier chapter on INSERT" throughout the book, we have started off by describing the SQL language globally, and then detailing each command group separately. It's a bit like a course for auto mechanics, say, you start off by first describing the layout of the car and all it's major parts such as the engine, the gearbox etc., before going on to discuss topics like the detailed construction of the engine.
Primarily, this book is designed to teach you how to use SQL to create, modify, maintain and use databases in practical situations. It is not intended to be an academic treatise on the subject, and so does not go into the mathematical basis of the topics considered. What it does contain is lots of examples and discussions on how they work. You should work your way through this book by reading through a section, and actually trying out each SQL query presented for yourself. If you do not have access to an SQL based database, then you can order a fully functional ANSI/ISO SQL database at an affordable price, by sending off the order form at the back of this book. The quickest and easiest method of learning SQL (or indeed any computer language) is to use it in real life, practical situations. The chapters of this book are laid out so that each section builds upon the information and examples presented in the previous chapters. By following the SQL query examples, you will create a database, populate it and then use it to retrieve information.
Remember that the SQL queries in this book are only given as examples. They represent one possible method of retrieving the results that you want. As you gain confidence in SQL, you may be able to construct a more elegant query to solve a problem than the one that we have used. This just goes to show the power and flexibility of SQL.
The structure of this book is such that as you progress through it, you will be exposed to more and more complex aspects of SQL. If you follow through the book, you will find that you are not suddenly presented with anything particularly difficult. Rather, you will be gradually lead through and actively encouraged to try out SQL queries and variations of queries until you have thoroughly understood the underlying ideas.
The chapters will not all take the same amount of time to read and understand. You will benefit most if you sit down, start at a new section, and work your way through until it is completed. Although we understand that you may find some of the longer sections difficult to finish in one session. You should nonetheless endeavour to complete each section in as few sittings as possible. Taking short breaks to think over concepts learned as you progress through the section is also a good idea as it reinforces the learning process. You should try to understand the underlying concepts of what you are learning rather than coasting through the book.
1.5.1 Notational conventions.
The following notational conventions are used throughout this book:
BOLD TYPE These are keywords and data in a statement. They are to appear exactly as they are shown in bold.
{ } Curly braces group together logically distinct sections of a command. If the braces are followed by an asterix (*), then the section inside them can occur zero or more times in a statement. If followed by a plus (+), then the section inside must appear at least once in the statement.
[ ] Square brackets are used to signify sections of a statement that are optional.
( ) Parentheses in bold are part of the SQL command, and must appear as shown. Parentheses which are not in bold are to indicate the logical order of evaluation.
... The ellipses show that the section immediately proceeding them may be repeated any number of times.
| The vertical bar means "or".
Throughout this , SQL command structure will be explained by using examples of actual statements.
FOR MORE INFORMATION VISIT THE FOLLOWING LINKS:
http://www.stat.berkeley.edu/~spector/sql.pdf

oriented features - and, since Oracle 10g, a grid-enabled database - the ability to scale across multiple inexpensive servers to provide more processing resources.
Each release of the Oracle database brings new features to improve scalability, reliability, performance and availability. We'll be examining some of these new features in later Oracle tutorials.
A relational database, therefore, can be regarded as containing a set of 2-dimensional tables ("relations") with each table comprising rows ("tuples") and columns ("domains"). Relationships between database tables are created when one table has a column with the same meaning as a column in another table. The actual values of the columns are irrelevant but they must refer to/mean the same thing.
Let's take the example of a very simple database with just 2 tables (tuples):
Now that we can say that these two tables are related, the other part of relational database model - relational calculus (which is essentially set theory) enables relations (i.e. database tables) to be combined in various ways:
http://www.java2s.com/Tutorial/Oracle/0020__Introduction/Catalog0020__Introduction.htm
http://w2.syronex.com/jmr/edu/db/
http://w2.syronex.com/jmr/edu/db/
http://www.exforsys.com/tutorials/oracle-apps.html

What is an Oracle database?
Oracle is an object-relational hybrid database - a relational database with added object-oriented features - and, since Oracle 10g, a grid-enabled database - the ability to scale across multiple inexpensive servers to provide more processing resources.
Each release of the Oracle database brings new features to improve scalability, reliability, performance and availability. We'll be examining some of these new features in later Oracle tutorials.
What is a relational database?
A British engineer Ted Codd working for IBM elucidated the theory of relational databases "A Relational Model of Data for Large Shared Data Banks" in the 1970s whilst working for IBM. At the core of this relational model is the concept of normalisation which is the separation of the logical and physical data models. This enables you to "see" the database in a completely different way to its underlying structure. The importance of this separation is that it makes relational databases extremely flexible unlike, for example, hierarchical databases. This flexibility means that either layer can be changed without affecting the other. With earlier database models, changes in business requirements requiring new data structures necessitated the complete re-design of the database.A relational database, therefore, can be regarded as containing a set of 2-dimensional tables ("relations") with each table comprising rows ("tuples") and columns ("domains"). Relationships between database tables are created when one table has a column with the same meaning as a column in another table. The actual values of the columns are irrelevant but they must refer to/mean the same thing.
Let's take the example of a very simple database with just 2 tables (tuples):
- employees
- departments
- employee_id
- employee_ name
- department_id
- department_id
- department_name
Now that we can say that these two tables are related, the other part of relational database model - relational calculus (which is essentially set theory) enables relations (i.e. database tables) to be combined in various ways:
- the union of 2 relations results in a set of data containing those elements that exist in one or other relation (or both relations);
- the result of the join (the intersection) of 2 relations is the set of elements that exist in both relations;
- the exclusive "OR" produces the set of items that are in either of the relations but not both
- an outer-join is the same as the join but also includes elements from one or both relations that are not in the other (depending on whether a full outer-join or a left or right outer join is performed)
- relations can also be subtracted from each other leaving the set of elements that were in the first relation but not the 2nd.
http://www.java2s.com/Tutorial/Oracle/0020__Introduction/Catalog0020__Introduction.htm
http://w2.syronex.com/jmr/edu/db/
http://w2.syronex.com/jmr/edu/db/
http://www.exforsys.com/tutorials/oracle-apps.html